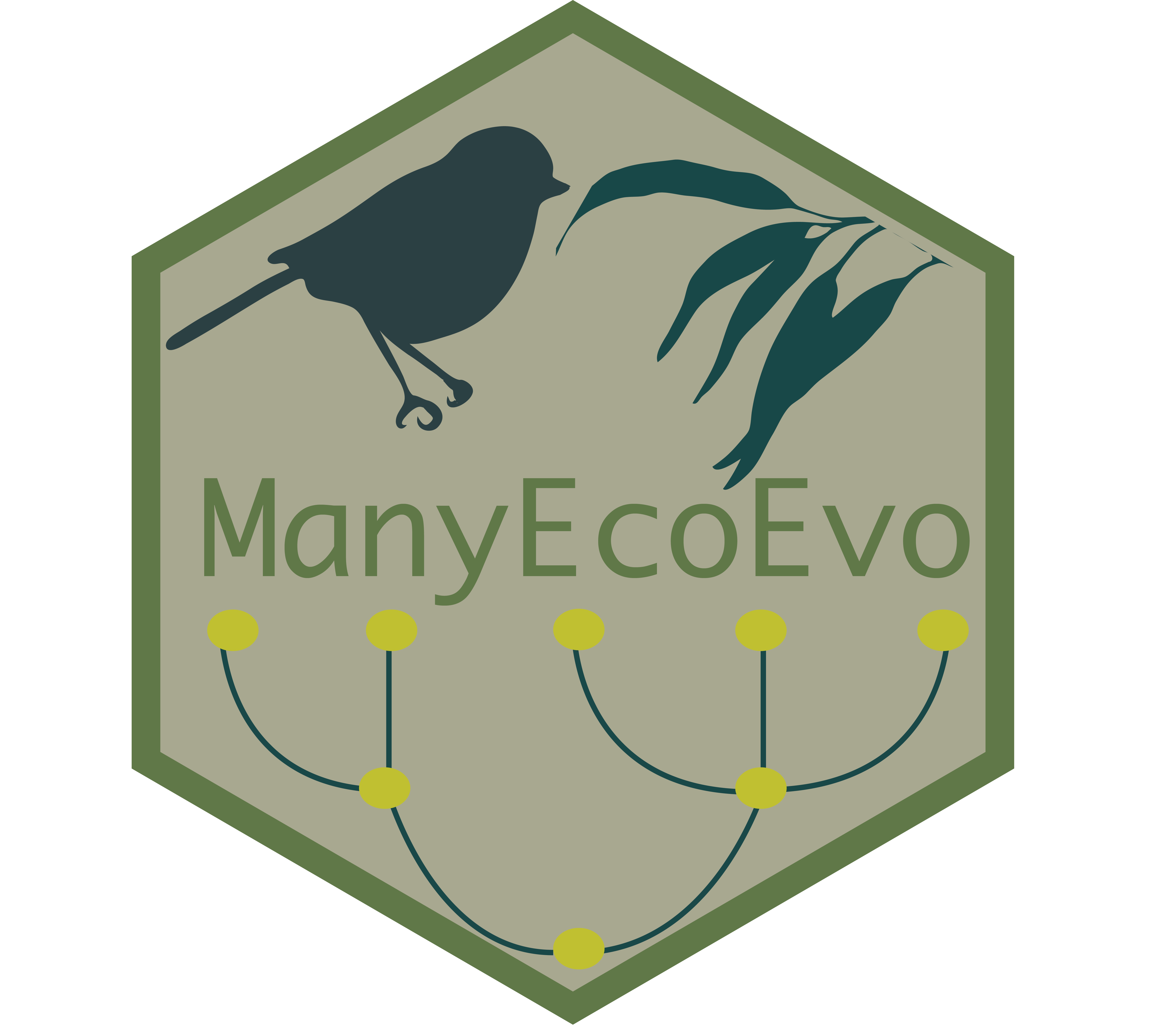
Exclude extreme estimates above a threshold parameter sd
Source:R/exclude_extreme_estimates.R
exclude_extreme_estimates.Rd
Exclude extreme estimates above a threshold parameter sd
Usage
exclude_extreme_estimates(
data,
outcome_variable,
outcome_SE,
sd_threshold = numeric(1L),
param_table,
.fn = ...,
...
)
Arguments
- data
A dataframe of analyst estimates
- outcome_variable
the name of the variable in
data
containing the analyst estimates- outcome_SE
variable in
data
containing analyst SE estimates- sd_threshold
A numeric threshold multiplyer see details
- param_table
A dataframe containing population parameters
mean
andsd
for eachvariable
in a givendataset
- .fn
An optional function that will transform parameter estimates to the same scale as
outcome_variable
indata
- ...
Arguments supplied to
.fn
Details
This function is used to exclude extreme estimates from a dataset. The function
calculates a threshold for exclusion based on the mean and standard deviation of
the population parameter estimates in param_table
. The threshold is calculated
as the mean of the population parameter plus sd_threshold
times the standard
deviation of the population parameter. Estimates in data
that are greater than
this threshold are excluded from the output.
If the user chooses to supply .fn
and ...
arguments, the function will transform
the population parameter estimates in param_table
to the same scale as the
outcome_variable
in data
using .fn
, before calculating the threshold for exclusion.
Examples
# example code
data <- ManyEcoEvo_yi %>%
mutate(data =
map_if(data,
~ filter(.x,
stringr::str_detect(response_variable_name,
"average.proportion.of.plots.containing",
negate = TRUE)),
.p = dataset == "eucalyptus")) %>%
mutate(
diversity_data =
map2(
.x = diversity_data,
.y = data,
.f = ~ semi_join(.x, .y, join_by(id_col)) %>%
distinct()
)
) %>%
prepare_response_variables(
estimate_type = "yi",
param_table =
ManyEcoEvo:::analysis_data_param_tables,
dataset_standardise = "blue tit",
dataset_log_transform = "eucalyptus") %>%
generate_yi_subsets() %>% #TODO: must be run after prepare_response_variables??
apply_VZ_exclusions(
VZ_colname = list("eucalyptus" = "se_log",
"blue tit" = "VZ"),
VZ_cutoff = 3) %>%
filter(dataset == "eucalyptus", estimate_type == "y25") %>%
pluck("data", 1)
#> Error in pluck(., "data", 1): could not find function "pluck"
sd_threshold = 3
param_table <- ManyEcoEvo:::analysis_data_param_tables
exclude_extreme_estimates(data, "mean_log", "se_log", 3, param_table, log_transform, estimate = mean, std.error = sd)
#>
#> ── Transforming `param_table` using `.fn`:
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#> ✔ Log-transformed out-of-sample predictions, using 10000 simulations.
#>
#> ── Excluding extreme estimates from data:
#> Error in UseMethod("left_join"): no applicable method for 'left_join' applied to an object of class "function"